Several years ago I'd written an article about screen savers in .NET. The very detailed article has since gone to PDF form (found here), but I thought it would be good to mention some of the finer points in case anyone else out there found this and was interested in writing a C#-based screen saver. The samples you'll generally find available aren't nearly good enough as they don't provide enough code, or the right code, to do the job.
In this case, I have a screen saver that mimics the screens seen in one of my favorite movies, "The Matrix." Here's the general idea:
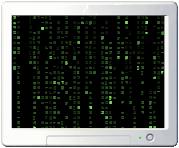
Aside from the actual simulation, which I'll leave to you to decipher from the code, two things are critical here. First, you have to properly accept (and handle) the command line parameters. And second, you have to deal with multiple screens. Here's some detail.
As for the command line, this is the code you'll need:
string cmd = args.Length > 0 ?
(args[0].Length > 1 ?
args[0].ToLower(CultureInfo.InvariantCulture).Trim().Substring(0, 2) :
args[0].ToLower(CultureInfo.InvariantCulture).Trim().Substring(0, 1)) : "";
if (cmd == "/c" || cmd == "-c" || cmd == "c" || cmd == "" )
{
// Options dialog.
} // if
else if (cmd == "/p" || cmd == "-p" || cmd == "p" )
{
// "Preview" mode.
} // else if
else if (cmd == "/s" || cmd == "-s" || cmd == "s" )
{
// Run screen saver.
} // else if
else
{
// Sorry...don't recognize the command line parameter.
} // else
The trickiest part is the "/c" option, which requires you to accept a window handle (as a string) and render the screen output there. (That's how the options dialog shows you the preview.) The details are in the code, which you can download here, but essentially you convert the handle from a string to an IntPtr, and then create a Graphics object from that. The option will come in this form:
/c:1269802
Multiple screens are another tricky aspect. The basic approach is you iterate the screens attached to the system, which you find in Screen.Allscreens, like so:
Int32 totWidth = 0;
Int32 totHeight = 0;
for (Int32 i = 0; i < Screen.AllScreens.Length; i++)
{
// Check the dimensions of this screen
if ( Screen.AllScreens[i].Bounds.X == 0 )
{
// We simply compare the width of this screen to
// the total width we've accumulated, and if greater,
// use this screen's width. Otherwise, we're covered.
if (Screen.AllScreens[i].Bounds.Width > totWidth )
totWidth = Screen.AllScreens[i].Bounds.Width;
} // if
else
{
// The widths are additive...
totWidth += Screen.AllScreens[i].Bounds.Width;
} // else
// Height
if (Screen.AllScreens[i].Bounds.Y == 0)
{
// We simply compare the height of this screen, similar
// to what we did for width.
if (Screen.AllScreens[i].Bounds.Height > totHeight)
totHeight = Screen.AllScreens[i].Bounds.Height;
} // if
else
{
// The heights are additive...
totHeight += Screen.AllScreens[i].Bounds.Height;
} // else
} // for
To install the screen saver, simply copy the Matrix.scr file to your hard drive and right-click. Then select Install from the list of available options (you can alternatively test it or configure it as well). The compiled image in the download is for all CPUs, but feel free to recompile it to match your own system. Be sure to compile a release build, however, so the streams aren't too slow!
2a880b8a-4d78-4cc6-812a-6de001e5fbe8|0|.0
Tags:
c#,
screen saver,
matrix,
animation,
p/invoke
Categories:
.NET General